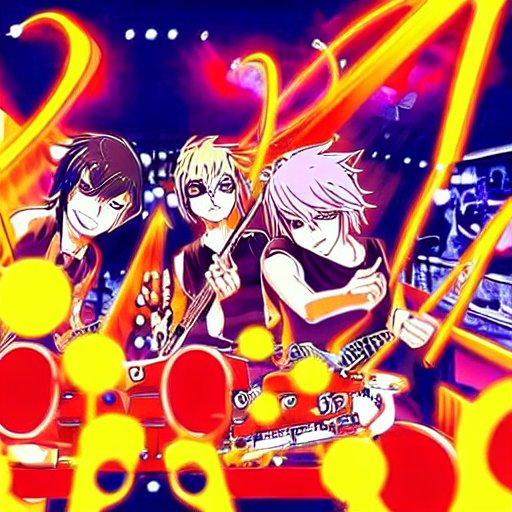
Image by Stable Diffusion.
Resources:
Exercise
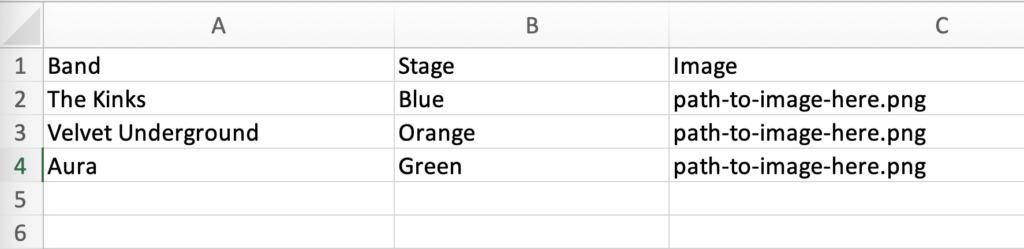
Enter some band names to a Spreadsheet. Here in Excel. Then convert the Excel to JSON:
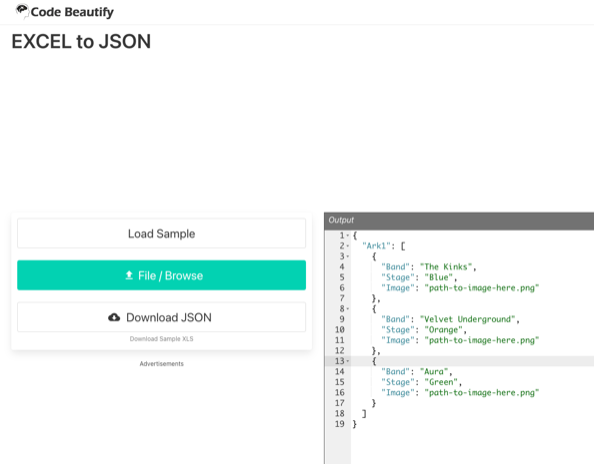
- Create a list of at least 5 real or imaginary bands in the form of a spreadsheet (Excell / Libre Office).
- If you need a JSON array, remember to mark i up like this:
[ ‘Track One’ , ‘Track Two’ , ‘Track Nine’ ] - Images must be links to images in your media library on your WordPress.
- Convert the spreadsheet to JSON. Download or copy the code.
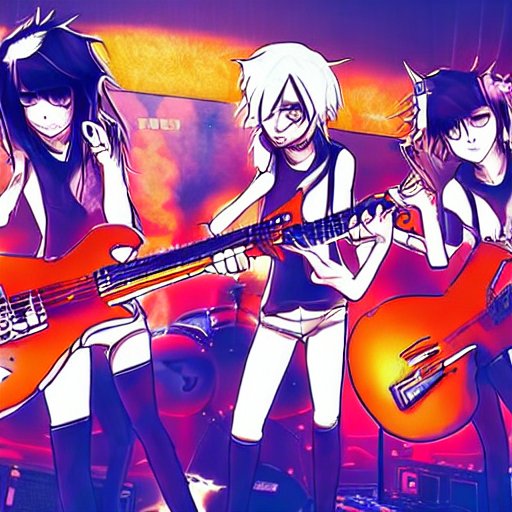
Modify the script so that the band list – or parts of it – will be displayed on your WP page.
Code: as JsFiddle
Now Create Your Band List
The bands object could have the following structure:
- Stage
- Name of the band.
- Picture / logo for the band (URI)
- Short description
- Tracks (as a list)
Add the code to WordPress
<div id="result"></div>
<script>
let bands = {
"Ark1": [
{
"Band": "The Kinks",
"Stage": "Blue",
"Image": "path-to-image-here.png"
},
{
"Band": "Velvet Underground",
"Stage": "Orange",
"Image": "path-to-image-here.png"
},
{
"Band": "Aura",
"Stage": "Green",
"Image": "path-to-image-here.png"
}
]
}
// loop out the list
for ( let i = 0; i<bands.Ark1.length; i++ ){
result.innerHTML += bands.Ark1[i].Band + "<br>"
}
</script>
And here is the result
By thine own ingenium continue …
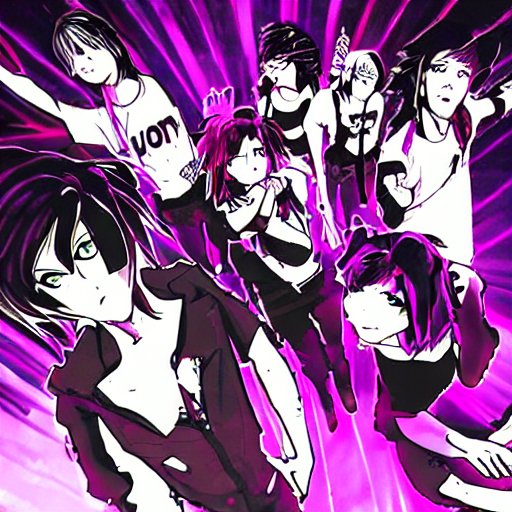
Now add real images. You can get the image URI in the WP Media Library. Also add famous songs by each band. Loop them out …
Fetch( url/file )
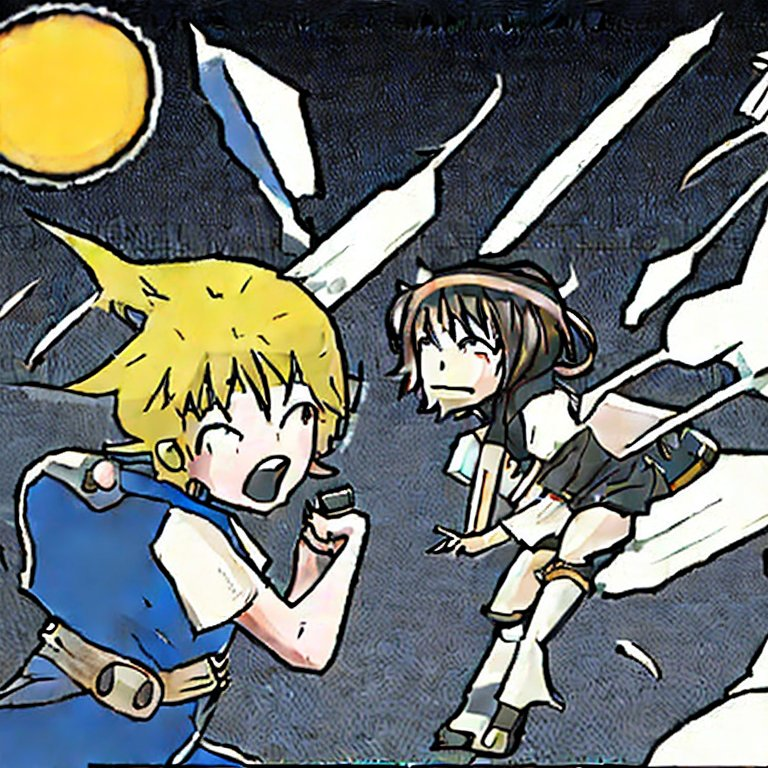
Probably it’s more convenient if your data is served as JSON from your server. Create a directory somwhere convenient for the JSON. In this sample we use the RAW data from a file on Github.
Google Sheets as a JSON endpoint
A spreadsheet may be used as a JSON “database”. Read this article.
Working Code Link
See this Gist on Github.